1.建立單一檔案上傳頁面(fileUpload.jsp):
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@ taglib prefix="s" uri="/struts-tags" %>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'fileUpload.jsp' starting page</title>
</head>
<body>
<font color="red"><s:actionerror/></font>
<font color="red"><s:fielderror/></font>
<form action="<%=path%>/fileUpload" method="post" enctype="multipart/form-data">
<table>
<tr>
<td>Title:</td>
<td><input type="text" name="title"></td>
</tr>
<tr>
<td>檔案名稱:</td>
<td><input type="file" name="upload"></td>
</tr>
<tr>
<td><input type="submit" value="上傳..."></td>
</tr>
</table>
</form>
</body>
</html>
2.建立上傳成功頁面(fileUpload_Success.jsp):
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib prefix="s" uri="/struts-tags" %> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'fileUpload_Success.jsp' starting page</title> </head> <body> FileUpload ok!!<br> Title:<s:property value="title"/><br> FullFileName:<s:property value="uploadFileName"/><br> FileContentType:<s:property value="uploadContentType"/><br> RealPath(Save):<s:property value="savePath"/> </body> </html>
3.建立上傳Action(FileUploadAction.java):
package com.action.test; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import org.apache.struts2.ServletActionContext; import com.opensymphony.xwork2.ActionSupport; public class FileUploadAction extends ActionSupport { /** * */ private static final long serialVersionUID = 1L; private File upload; private String uploadContentType; private String uploadFileName; private String title; private String savePath; private String targetDir; public File getUpload() { return upload; } public void setUpload(File upload) { this.upload = upload; } public String getUploadContentType() { return uploadContentType; } public void setUploadContentType(String uploadContentType) { this.uploadContentType = uploadContentType; } public String getUploadFileName() { return uploadFileName; } public void setUploadFileName(String uploadFileName) { this.uploadFileName = uploadFileName; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getSavePath() { this.savePath = ServletActionContext.getServletContext().getRealPath(getTargetDir()); return savePath; } public void setSavePath(String savePath) { this.savePath = savePath; } public String getTargetDir() { return targetDir; } public void setTargetDir(String targetDir) { this.targetDir = targetDir; } @Override public String execute() throws Exception { return doUpload(); } public String doUpload(){ if(upload==null){ this.addActionError("請選擇檔案!!"); return INPUT; } //String fileName = this.upload.getName(); String path = this.upload.getPath(); try { FileInputStream in = new FileInputStream(path); FileOutputStream os = new FileOutputStream(this.getSavePath()+"\\"+this.uploadFileName); //System.out.println("os:"+path); //System.out.println("in:"+fileName); try { byte[] b = new byte[1024]; int len=0; while((len=in.read(b))>0){ os.write(b,0,len); } os.close(); in.close(); } catch (IOException e) { e.printStackTrace(); } } catch (FileNotFoundException e) { e.printStackTrace(); } return SUCCESS; } }
4.於struts.xml(src目錄下)加入Struts2 Action:
<action name="fileUpload" class="com.test.action.fileUpload.FileUploadAction"> <result>fileUpload_Success.jsp</result> <result name="input">fileUpload.jsp</result> <param name="targetDir">\\upload</param> </action>
若需限制每次檔案最大可上傳之大小,可加入下面參數於struts.xml內:
<!-- 一次最大上傳之檔案大小 --> <constant name="struts.multipart.maxSize" value="10240000"></constant>
指定上傳之暫存檔之目錄,可加入下面參數於struts.xml內:
<!-- temp dir --> <constant name="struts.multipart.saveDir" value="C:\\Program Files (x86)\\Apache Software Foundation\\Tomcat 6.0\\webapps\\Demo\\upload"></constant>
5.測試結果:
http://localhost:8080/Demo/test/fileUpload.jsp
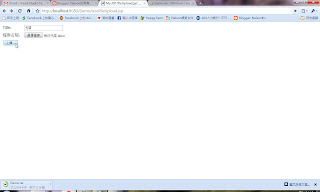
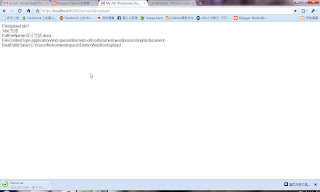
看到此畫面,恭喜您實作Struts2單一檔案上傳範例成功!
沒有留言:
張貼留言